# Material Design CSS Framework
[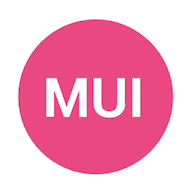](https://www.muicss.com)
MUI is a lightweight CSS framework that follows Google's Material Design guidelines.
[](https://gitter.im/muicss/mui?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge&utm_content=badge)
[](https://travis-ci.org/muicss/mui)
[](https://david-dm.org/muicss/mui)
[](https://david-dm.org/muicss/mui#info=devDependencies)
## Introduction
The MUI NPM package makes it easy to import MUI into a project and create a custom build that only includes the components you need.
The simplest way to use MUI is via the top level imports `muicss` and `muicss/react`:
```javascript
import { Appbar, Button, Panel } from 'muicss/react';
```
You can also optimize your builds by importing modules one-by-one from the lower level API:
```javascript
import Appbar from 'muicss/lib/react/appbar';
import Button from 'muicss/lib/react/button';
import Container from 'muicss/lib/react/container';
```
Here's an example of how to use MUI in a React app:
```javascript
import React from 'react';
import ReactDOM from 'react-dom';
import { Appbar, Button, Panel } from 'muicss/react';
class Example extends React.Component {
onClick() {
console.log('clicked on button');
}
render() {
return (
);
}
}
ReactDOM.render(, document.getElementById('example'));
```
## API Documentation
### React Library
All of the MUI React components can be accessed as top-level attributes of the `muicss/react` package. In addition, they can be accessed individually at `muicss/lib/react/{component}`.
#### Appbar
```jsx
import Appbar from 'muicss/lib/react/appbar';
```
Read more: https://www.muicss.com/docs/v1/react/appbar
#### Button
```jsx
import Button from 'muicss/lib/react/button';
* {String} color=default|primary|danger|accent
* {String} size=default|small|large
* {String} type=submit|button
* {String} variant=default|flat|raised|fab
* {Boolean} disabled=false|true
```
Read more: https://www.muicss.com/docs/v1/react/buttons
#### Checkbox
```jsx
import Checkbox from 'muicss/lib/react/checkbox';
* {String} label
* {String} value
* {Boolean} checked
* {Boolean} defaultChecked
* {Boolean} disabled=false|true
```
Read more: https://www.muicss.com/docs/v1/react/forms
#### Container
```jsx
import Container from 'muicss/lib/react/container';
* {Boolean} fluid=false|true
```
Read more: https://www.muicss.com/docs/v1/react/container
#### Divider
```jsx
import Divider from 'muicss/lib/react/divider';
```
Read more: https://www.muicss.com/docs/v1/react/dividers
#### Dropdown Component
##### Dropdown
```jsx
import Dropdown from 'muicss/lib/react/dropdown';
* {String or ReactElement} label
* {String} alignMenu=left|right
* {String} color=default|primary|danger|accent
* {String} size=default|small|large
* {String} variant=default|flat|raised|fab
* {Boolean} disabled
* {Function} onClick
* {Function} onSelect
```
Read more: https://www.muicss.com/docs/v1/react/dropdowns
##### DropdownItem
```jsx
import DropdownItem 'muicss/lib/react/dropdown-item';
* {String} link
* {String} target
* {String} value
* {Function} onClick
```
Read more: https://www.muicss.com/docs/v1/react/dropdowns
#### Form
```jsx
import Form from 'muicss/lib/react/form';
* {Boolean} inline=false|true
```
Read more: https://www.muicss.com/docs/v1/react/forms
#### Grid Elements
##### Row
```jsx
import Row from 'muicss/lib/react/row';
```
Read more: https://www.muicss.com/docs/v1/react/grid
##### Col
```jsx
import Col from 'muicss/lib/react/col';